Create and Set Tags like wordpress insert into a Database and Search by Tag
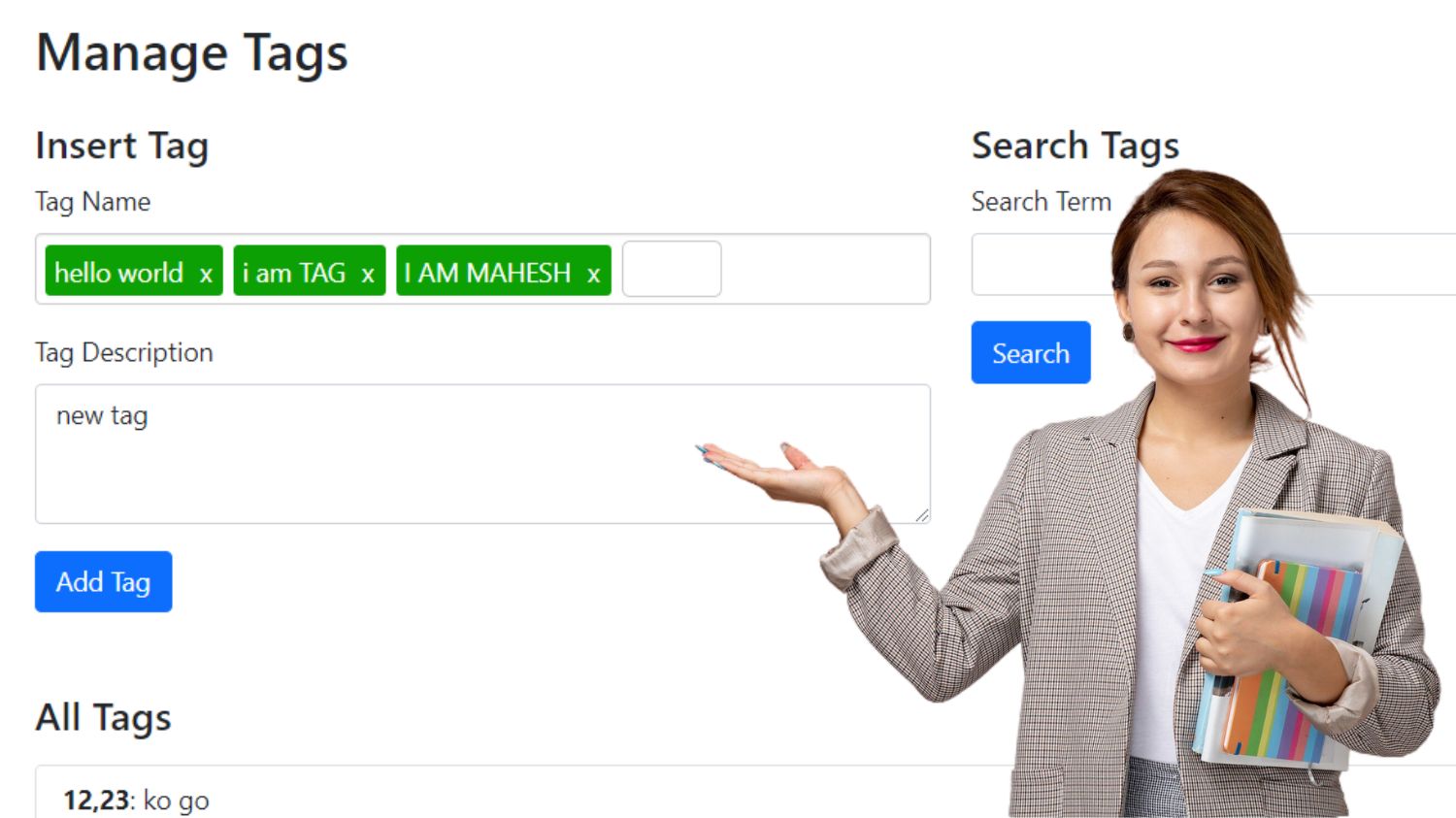
Tags are crucial in organizing website content, helping users easily navigate through information. Understanding how to create and set tags, like WordPress, insert them into a database, and search by tag efficiently can significantly improve the user experience. In this blog post, we will delve deeper into these topics, providing you with valuable insights and practical tips.
Setting Tags like WordPress
Setting tags on your website, similar to how it’s done on WordPress, involves categorizing content under specific keywords or phrases. This not only helps in organizing your content but also enhances the visibility of your posts to users. To effectively set tags, follow these steps:
- Identify relevant keywords or phrases that best describe your content
- Assign these tags to your posts or articles accordingly
- Ensure consistency in tagging across your website for easy accessibility
To create a tag management feature with name and description fields and a search option by tag, you can follow these steps:
- Database Setup:
Create a MySQL database and a table to store the tags with name and description
0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
CREATE DATABASE tag_db; USE tag_db; CREATE TABLE tags ( id INT AUTO_INCREMENT PRIMARY KEY, tag_name VARCHAR(255) NOT NULL, tag_description TEXT NOT NULL ); |
2. PHP Script for Tag Management: This script will handle the tag creation, display, and search functionality.
0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 |
<?php // Database connection details $servername = "localhost"; $username = "root"; $password = ""; // Replace with your database password $dbname = "tag_db"; // Create connection $conn = new mysqli($servername, $username, $password, $dbname); // Check connection if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } // Insert tag if ($_SERVER["REQUEST_METHOD"] == "POST" && isset($_POST['insert'])) { $tag_name = $_POST['tag_name']; $tag_description = $_POST['tag_description']; // Check if the tag already exists $check_sql = "SELECT * FROM tags WHERE tag_name = '$tag_name'"; $check_result = $conn->query($check_sql); if ($check_result->num_rows == 0) { $sql = "INSERT INTO tags (tag_name, tag_description) VALUES ('$tag_name', '$tag_description')"; if ($conn->query($sql) === TRUE) { echo "<div class='alert alert-success'>New tag created successfully</div>"; } else { echo "<div class='alert alert-danger'>Error: " . $sql . "<br>" . $conn->error . "</div>"; } } else { echo "<div class='alert alert-warning'>Tag already exists</div>"; } } // Search tags $search_results = []; if ($_SERVER["REQUEST_METHOD"] == "POST" && isset($_POST['search'])) { $search_term = $_POST['search_term']; $sql = "SELECT * FROM tags WHERE tag_name LIKE '%$search_term%'"; $result = $conn->query($sql); if ($result->num_rows > 0) { while ($row = $result->fetch_assoc()) { $search_results[] = $row; } } else { echo "<div class='alert alert-info'>No results found</div>"; } } // Get all tags $tags = []; $sql = "SELECT * FROM tags"; $result = $conn->query($sql); if ($result->num_rows > 0) { while ($row = $result->fetch_assoc()) { $tags[] = $row; } } $conn->close(); ?> <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Tag Management</title> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.1.3/dist/css/bootstrap.min.css" rel="stylesheet"> <link href="https://cdnjs.cloudflare.com/ajax/libs/bootstrap-tagsinput/0.8.0/bootstrap-tagsinput.css" rel="stylesheet"> <style> .bootstrap-tagsinput { width: 100%; } .bootstrap-tagsinput .tag { background-color: #add8e6; border: 1px solid #add8e6; padding: 5px; border-radius: 3px; margin-right: 2px; } </style> </head> <body> <div class="container mt-5"> <h2 class="mb-4">Manage Tags</h2> <div class="row"> <div class="col-md-6"> <h4>Insert Tag</h4> <form method="POST" action=""> <div class="mb-3"> <label for="tag_name" class="form-label">Tag Name</label> <input type="text" class="form-control" id="tag_name" name="tag_name" data-role="tagsinput"> </div> <div class="mb-3"> <label for="tag_description" class="form-label">Tag Description</label> <textarea class="form-control" id="tag_description" name="tag_description" rows="3"></textarea> </div> <button type="submit" name="insert" class="btn btn-primary">Add Tag</button> </form> </div> <div class="col-md-6"> <h4>Search Tags</h4> <form method="POST" action=""> <div class="mb-3"> <label for="search_term" class="form-label">Search Term</label> <input type="text" class="form-control" id="search_term" name="search_term"> </div> <button type="submit" name="search" class="btn btn-primary">Search</button> </form> <?php if (!empty($search_results)): ?> <h4 class="mt-4">Search Results:</h4> <ul class="list-group"> <?php foreach ($search_results as $result): ?> <li class="list-group-item"> <strong><?php echo htmlspecialchars($result['tag_name']); ?></strong>: <?php echo htmlspecialchars($result['tag_description']); ?> </li> <?php endforeach; ?> </ul> <?php endif; ?> </div> </div> <h4 class="mt-5">All Tags</h4> <ul class="list-group mt-3"> <?php foreach ($tags as $tag): ?> <li class="list-group-item"> <strong><?php echo htmlspecialchars($tag['tag_name']); ?></strong>: <?php echo htmlspecialchars($tag['tag_description']); ?> </li> <?php endforeach; ?> </ul> </div> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.1.3/dist/js/bootstrap.bundle.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/bootstrap-tagsinput/0.8.0/bootstrap-tagsinput.min.js"></script> </body> </html> |
Explanation:
- Database Connection: The PHP script establishes a connection to the MySQL database.
- Insert Tag: When the form for inserting a tag is submitted, the script checks if the tag name already exists. If not, it inserts the tag name and description into the
tags
table. - Search Tags: When the form is submitted, the script executes a query to find tags that match the search term.
- Retrieve All Tags: The script retrieves all tags from the database and displays them in a list.
- HTML Structure: The form uses Bootstrap for styling and includes fields for entering tag name and descriptions. The search functionality allows users to search for tags by name.